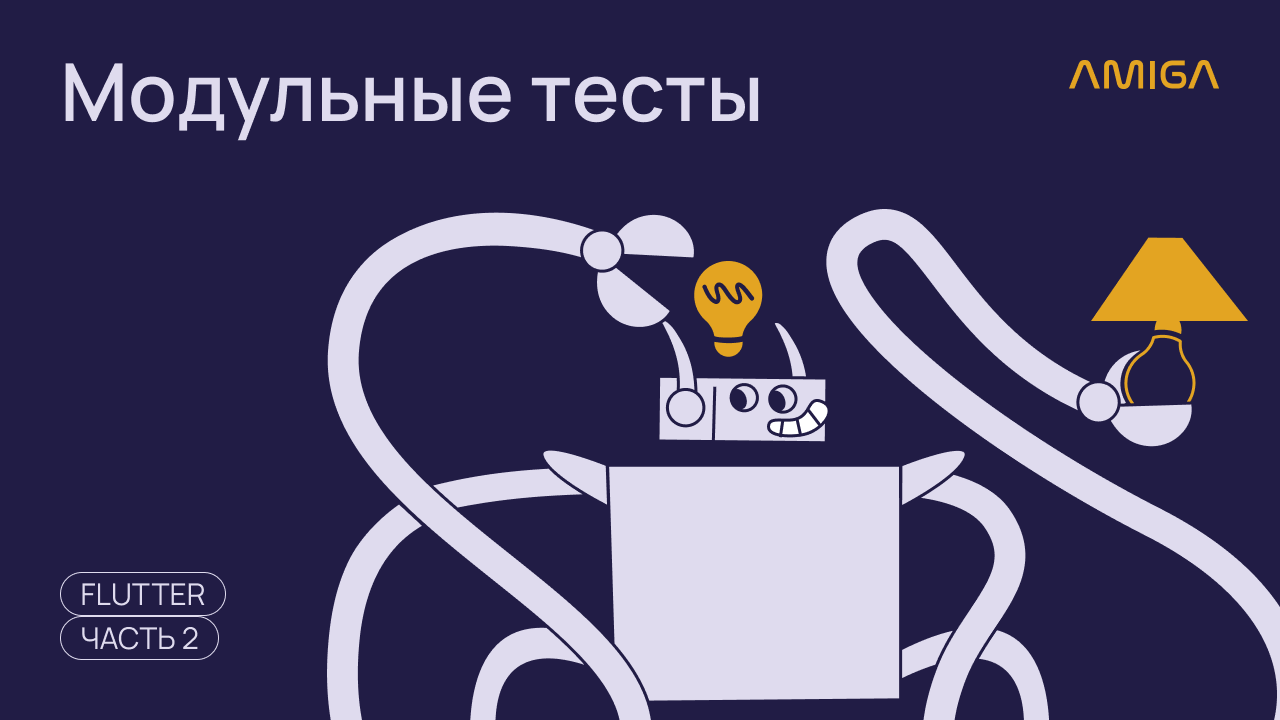
class Validator {
static bool validateEmail(String value) {
return value.isNotEmpty;
}
static bool validatePassword(String value) {
return value.isNotEmpty;
}
}
extension StringExtension on String? {
bool get isNullOrEmpty => this == null || this!.isEmpty;
}
import 'package:testing_examples/part2/ext/extension.dart';
import 'package:testing_examples/part2/util/utils.dart';
bool login(String? email, String? password) {
if (email.isNullOrEmpty || password.isNullOrEmpty) {
return false;
}
return Validator.validateEmail(email!) && Validator.validatePassword(password!);
}
import 'package:flutter_test/flutter_test.dart';
void main() {
}
Для того, чтобы создать Unit-тест, используем функцию test, передавая ей 2 параметра: description и body.
void main() {
test('validateEmail should return true when the email is not empty', () {
// body
});
}
test('validateEmail should return true when the email is not empty', () {
// Arrange
String validEmail = 'test@example.com';
// Act
bool result = Validator.validateEmail(validEmail);
// Assert
expect(result, true);
});
test('validateEmail should return false when the email is empty', () {
// Arrange
String invalidEmail = '';
// Act
bool result = Validator.validateEmail(invalidEmail);
// Assert
expect(result, false);
});
test('validatePassword should return true when the password is not empty', () {
// Arrange
String validPassword = 'password123';
// Act
bool result = Validator.validatePassword(validPassword);
// Assert
expect(result, true);
});
test('validatePassword should return false when the password is empty', () {
// Arrange
String invalidPassword = '';
// Act
bool result = Validator.validatePassword(invalidPassword);
// Assert
expect(result, false);
});
group('validateEmail', () {
test('validateEmail should return true when the email is not empty', () {
// body
});
test('validateEmail should return false when the email is empty', () {
// body
});
});
group('validatePassword', () {
test('validatePassword should return true when the password is not empty',
// body
});
test('validatePassword should return false when the password is empty', () {
// body
});
});
group('login', () {
test('login should return false when the email is empty', () {
// Arrange
String? email;
String password = 'password123';
// Act
bool result = login(email, password);
// Assert
expect(result, false);
});
test('login should return false when the password is empty', () {
// Arrange
String email = 'ntminh@gmail.vn';
String? password;
// Act
bool result = login(email, password);
// Assert
expect(result, false);
});
test('login should return false when the email and password are empty', () {
// Arrange
String? email;
String? password;
// Act
bool result = login(email, password);
// Assert
expect(result, false);
});
test('login should return true when the email and password are not empty',
() {
// Arrange
String email = 'ntminh@gmail.vn';
String password = 'password123';
// Act
bool result = login(email, password);
// Assert
expect(result, true);
});
});
expect(actual, matcher);